Drop-down menu
The Dcat\Admin\Widgets\Dropdown
class is a quick way to help you build dropdown menu functions.
Basic Usage
<?php
use Dcat\Admin\Widgets\Dropdown;
use Dcat\Admin\Layout\Content;
class MyController
{
public function index(Content $content)
{
$options = [
'Name 1',
'Name 2',
'Name 3',
'Name 4',
'Name 5',
];
$dropdown = Dropdown::make($options)
->button('Category Navigation') // Set button
->buttonClass('btn btn-white waves-effect') // Set the button style
->map(function ($label, $key) {
// Format menu options
$url = admin_url('categories/'.$key);
return "<a href='$url'>{$label}</a>";
});
return $content->body($dropdown);
}
}
result
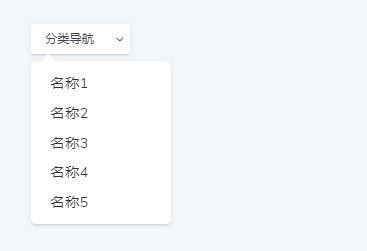
Click on the menu to change button text
The click
method allows the text of the selected menu to be displayed in the button, similar to the result of a drop-down selection.
$options = [
...
];
$dropdown = Dropdown::make($options)
->button('option') // Set button
->click();
Set TITLE
$options1 = [
'Name 1',
'Name 2',
];
$options2 = [
'Test 1',
'Test 2',
];
$dropdown = Dropdown::make()
->button('Use of TITLE')
->options($options1, 'TITLE1')
->options($options2, 'TITLE2');
result
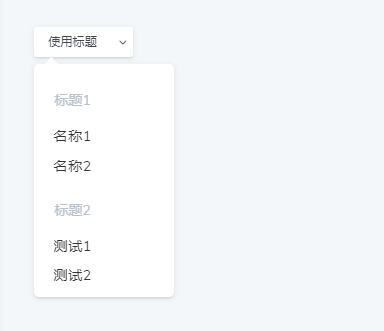
增加分割线
$options = [
'Name 1',
'Name 2',
Dropdown::DIVIDER,
'Name 3',
'Name 4',
];
$dropdown = Dropdown::make()
->button('Using dividing lines')
->options($options)
result
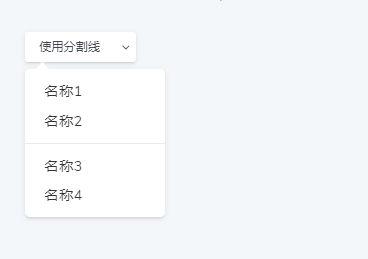
Custom buttons
public function index(Content $content)
{
$options = [
'Name 1',
'Name 2',
'Name 3',
'Name 4',
'Name 5',
];
$dropdown = Dropdown::make($options)
->map(function ($label, $key) {
// Formatting menu options
$url = admin_url('categories/'.$key);
return "<a href='$url'>{$label}</a>";
});
return $content->body(
<<<HTML
<div class='dropdown'>
<button class='btn btn-primary dropdown-toggle' data-toggle='dropdown'>
<i class='feather icon-email'></i> Custom buttons
</button>
{$dropdown->render()}
</div>
HTML
);
}
result
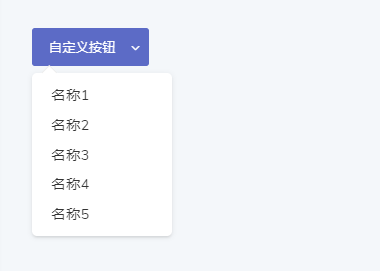